注意
转到末尾下载完整的示例代码。
颜色图归一化#
演示如何使用 norm 以非线性方式将颜色图映射到数据上。
import matplotlib.pyplot as plt
import numpy as np
import matplotlib.colors as colors
N = 100
LogNorm#
此示例数据有一个低峰,其中心有一个尖峰。如果使用线性颜色刻度绘制,则只会看到尖峰。要同时看到驼峰和尖峰,则需要 z/颜色轴上的对数刻度。
不是使用 pcolor(log10(Z))
变换数据,而是可以使用 LogNorm
使颜色映射呈对数形式。
X, Y = np.mgrid[-3:3:complex(0, N), -2:2:complex(0, N)]
Z1 = np.exp(-X**2 - Y**2)
Z2 = np.exp(-(X * 10)**2 - (Y * 10)**2)
Z = Z1 + 50 * Z2
fig, ax = plt.subplots(2, 1)
pcm = ax[0].pcolor(X, Y, Z, cmap='PuBu_r', shading='nearest')
fig.colorbar(pcm, ax=ax[0], extend='max', label='linear scaling')
pcm = ax[1].pcolor(X, Y, Z, cmap='PuBu_r', shading='nearest',
norm=colors.LogNorm(vmin=Z.min(), vmax=Z.max()))
fig.colorbar(pcm, ax=ax[1], extend='max', label='LogNorm')
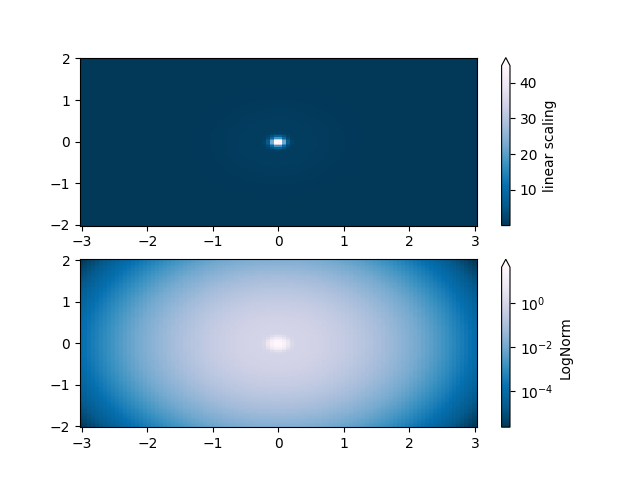
PowerNorm#
此示例数据混合了 X 中的幂律趋势和 Y 中的整流正弦波。如果使用线性颜色刻度绘制,则 X 中的幂律趋势会部分遮盖 Y 中的正弦波。
可以使用 PowerNorm
消除幂律。
X, Y = np.mgrid[0:3:complex(0, N), 0:2:complex(0, N)]
Z = (1 + np.sin(Y * 10)) * X**2
fig, ax = plt.subplots(2, 1)
pcm = ax[0].pcolormesh(X, Y, Z, cmap='PuBu_r', shading='nearest')
fig.colorbar(pcm, ax=ax[0], extend='max', label='linear scaling')
pcm = ax[1].pcolormesh(X, Y, Z, cmap='PuBu_r', shading='nearest',
norm=colors.PowerNorm(gamma=0.5))
fig.colorbar(pcm, ax=ax[1], extend='max', label='PowerNorm')
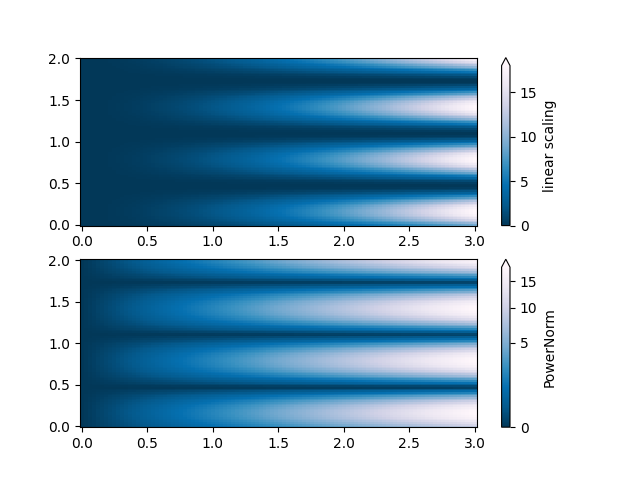
SymLogNorm#
此示例数据有两个驼峰,一个负驼峰和一个正驼峰。正驼峰的幅度是负驼峰的 5 倍。如果使用线性颜色刻度绘制,则负驼峰中的细节将被遮盖。
在这里,我们使用 SymLogNorm
将正负数据分别以对数方式缩放。
请注意,颜色栏标签看起来不太好。
X, Y = np.mgrid[-3:3:complex(0, N), -2:2:complex(0, N)]
Z1 = np.exp(-X**2 - Y**2)
Z2 = np.exp(-(X - 1)**2 - (Y - 1)**2)
Z = (5 * Z1 - Z2) * 2
fig, ax = plt.subplots(2, 1)
pcm = ax[0].pcolormesh(X, Y, Z, cmap='RdBu_r', shading='nearest',
vmin=-np.max(Z))
fig.colorbar(pcm, ax=ax[0], extend='both', label='linear scaling')
pcm = ax[1].pcolormesh(X, Y, Z, cmap='RdBu_r', shading='nearest',
norm=colors.SymLogNorm(linthresh=0.015,
vmin=-10.0, vmax=10.0, base=10))
fig.colorbar(pcm, ax=ax[1], extend='both', label='SymLogNorm')
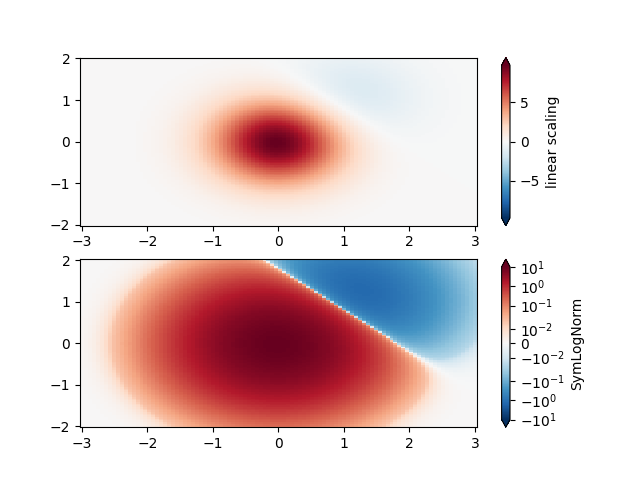
自定义 Norm#
或者,可以使用自定义归一化来缩放上述示例数据。这种方法以不同于正数据的方式对负数据进行归一化。
# Example of making your own norm. Also see matplotlib.colors.
# From Joe Kington: This one gives two different linear ramps:
class MidpointNormalize(colors.Normalize):
def __init__(self, vmin=None, vmax=None, midpoint=None, clip=False):
self.midpoint = midpoint
super().__init__(vmin, vmax, clip)
def __call__(self, value, clip=None):
# I'm ignoring masked values and all kinds of edge cases to make a
# simple example...
x, y = [self.vmin, self.midpoint, self.vmax], [0, 0.5, 1]
return np.ma.masked_array(np.interp(value, x, y))
fig, ax = plt.subplots(2, 1)
pcm = ax[0].pcolormesh(X, Y, Z, cmap='RdBu_r', shading='nearest',
vmin=-np.max(Z))
fig.colorbar(pcm, ax=ax[0], extend='both', label='linear scaling')
pcm = ax[1].pcolormesh(X, Y, Z, cmap='RdBu_r', shading='nearest',
norm=MidpointNormalize(midpoint=0))
fig.colorbar(pcm, ax=ax[1], extend='both', label='Custom norm')
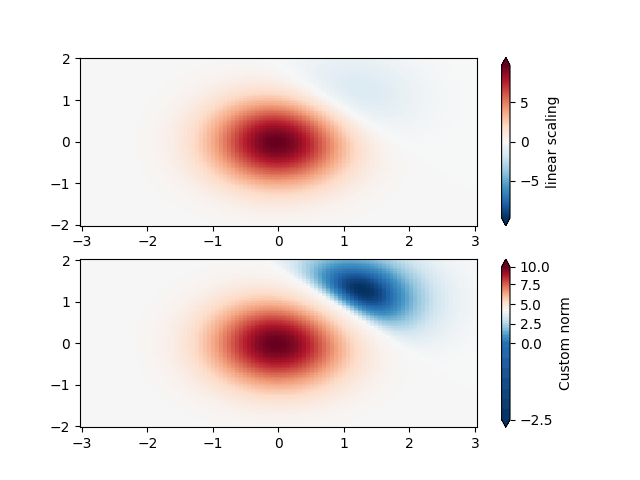
BoundaryNorm#
为了任意划分颜色刻度,可以使用 BoundaryNorm
;通过为颜色提供边界,此 norm 将第一个颜色放在第一对之间,第二个颜色放在第二对之间,依此类推。
fig, ax = plt.subplots(3, 1, layout='constrained')
pcm = ax[0].pcolormesh(X, Y, Z, cmap='RdBu_r', shading='nearest',
vmin=-np.max(Z))
fig.colorbar(pcm, ax=ax[0], extend='both', orientation='vertical',
label='linear scaling')
# Evenly-spaced bounds gives a contour-like effect.
bounds = np.linspace(-2, 2, 11)
norm = colors.BoundaryNorm(boundaries=bounds, ncolors=256)
pcm = ax[1].pcolormesh(X, Y, Z, cmap='RdBu_r', shading='nearest',
norm=norm)
fig.colorbar(pcm, ax=ax[1], extend='both', orientation='vertical',
label='BoundaryNorm\nlinspace(-2, 2, 11)')
# Unevenly-spaced bounds changes the colormapping.
bounds = np.array([-1, -0.5, 0, 2.5, 5])
norm = colors.BoundaryNorm(boundaries=bounds, ncolors=256)
pcm = ax[2].pcolormesh(X, Y, Z, cmap='RdBu_r', shading='nearest',
norm=norm)
fig.colorbar(pcm, ax=ax[2], extend='both', orientation='vertical',
label='BoundaryNorm\n[-1, -0.5, 0, 2.5, 5]')
plt.show()
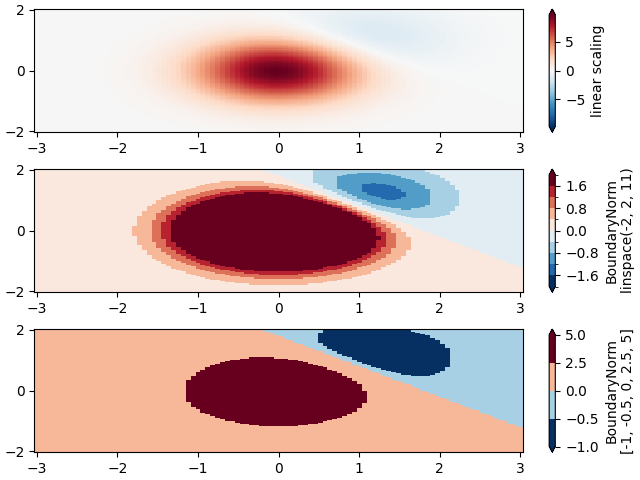
脚本的总运行时间:(0 分钟 8.699 秒)