注意
转到末尾下载完整的示例代码。
括号箭头上的角度注释#
此示例演示如何将角度注释添加到使用 FancyArrowPatch
创建的括号箭头样式。angleA 和 angleB 从垂直线测量,向左为正(向右为负)。蓝色 FancyArrowPatch
箭头表示 angleA 和 angleB 从垂直方向出发的方向,轴文本注释角度大小。
import matplotlib.pyplot as plt
import numpy as np
from matplotlib.patches import FancyArrowPatch
def get_point_of_rotated_vertical(origin, line_length, degrees):
"""Return xy coordinates of the vertical line end rotated by degrees."""
rad = np.deg2rad(-degrees)
return [origin[0] + line_length * np.sin(rad),
origin[1] + line_length * np.cos(rad)]
fig, ax = plt.subplots()
ax.set(xlim=(0, 6), ylim=(-1, 5))
ax.set_title("Orientation of the bracket arrows relative to angleA and angleB")
style = ']-['
for i, angle in enumerate([-40, 0, 60]):
y = 2*i
arrow_centers = ((1, y), (5, y))
vlines = ((1, y + 0.5), (5, y + 0.5))
anglesAB = (angle, -angle)
bracketstyle = f"{style}, angleA={anglesAB[0]}, angleB={anglesAB[1]}"
bracket = FancyArrowPatch(*arrow_centers, arrowstyle=bracketstyle,
mutation_scale=42)
ax.add_patch(bracket)
ax.text(3, y + 0.05, bracketstyle, ha="center", va="bottom", fontsize=14)
ax.vlines([line[0] for line in vlines], [y, y], [line[1] for line in vlines],
linestyles="--", color="C0")
# Get the top coordinates for the drawn patches at A and B
patch_tops = [get_point_of_rotated_vertical(center, 0.5, angle)
for center, angle in zip(arrow_centers, anglesAB)]
# Define the connection directions for the annotation arrows
connection_dirs = (1, -1) if angle > 0 else (-1, 1)
# Add arrows and annotation text
arrowstyle = "Simple, tail_width=0.5, head_width=4, head_length=8"
for vline, dir, patch_top, angle in zip(vlines, connection_dirs,
patch_tops, anglesAB):
kw = dict(connectionstyle=f"arc3,rad={dir * 0.5}",
arrowstyle=arrowstyle, color="C0")
ax.add_patch(FancyArrowPatch(vline, patch_top, **kw))
ax.text(vline[0] - dir * 0.15, y + 0.7, f'{angle}°', ha="center",
va="center")
plt.show()
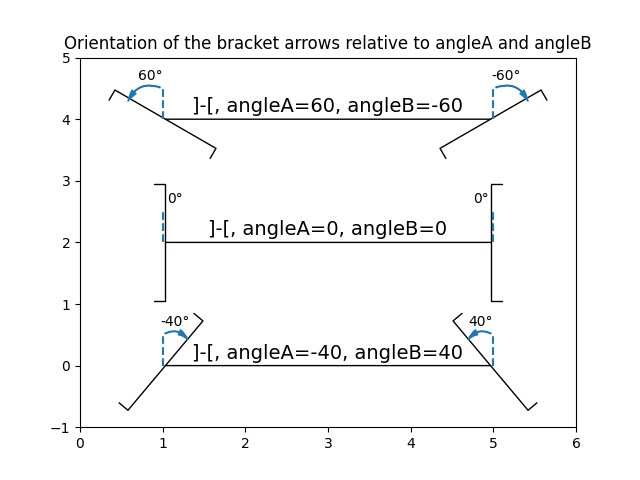