注意
转到结尾以下载完整的示例代码。
单选按钮#
使用单选按钮来选择绘图的属性。
单选按钮允许您在可视化中选择多个选项。 在这种情况下,这些按钮允许用户选择在图中显示的三个不同的正弦波之一。
可以使用label_props和radio_props参数来设置单选按钮的样式,每个参数都使用一个字典,其中包含艺术家属性名称的键和与按钮数量匹配的设置列表的值。
import matplotlib.pyplot as plt
import numpy as np
from matplotlib.widgets import RadioButtons
t = np.arange(0.0, 2.0, 0.01)
s0 = np.sin(2*np.pi*t)
s1 = np.sin(4*np.pi*t)
s2 = np.sin(8*np.pi*t)
fig, ax = plt.subplot_mosaic(
[
['main', 'freq'],
['main', 'color'],
['main', 'linestyle'],
],
width_ratios=[5, 1],
layout='constrained',
)
l, = ax['main'].plot(t, s0, lw=2, color='red')
radio_background = 'lightgoldenrodyellow'
ax['freq'].set_facecolor(radio_background)
radio = RadioButtons(ax['freq'], ('1 Hz', '2 Hz', '4 Hz'),
label_props={'color': 'cmy', 'fontsize': [12, 14, 16]},
radio_props={'s': [16, 32, 64]})
def hzfunc(label):
hzdict = {'1 Hz': s0, '2 Hz': s1, '4 Hz': s2}
ydata = hzdict[label]
l.set_ydata(ydata)
fig.canvas.draw()
radio.on_clicked(hzfunc)
ax['color'].set_facecolor(radio_background)
radio2 = RadioButtons(
ax['color'], ('red', 'blue', 'green'),
label_props={'color': ['red', 'blue', 'green']},
radio_props={
'facecolor': ['red', 'blue', 'green'],
'edgecolor': ['darkred', 'darkblue', 'darkgreen'],
})
def colorfunc(label):
l.set_color(label)
fig.canvas.draw()
radio2.on_clicked(colorfunc)
ax['linestyle'].set_facecolor(radio_background)
radio3 = RadioButtons(ax['linestyle'], ('-', '--', '-.', ':'))
def stylefunc(label):
l.set_linestyle(label)
fig.canvas.draw()
radio3.on_clicked(stylefunc)
plt.show()
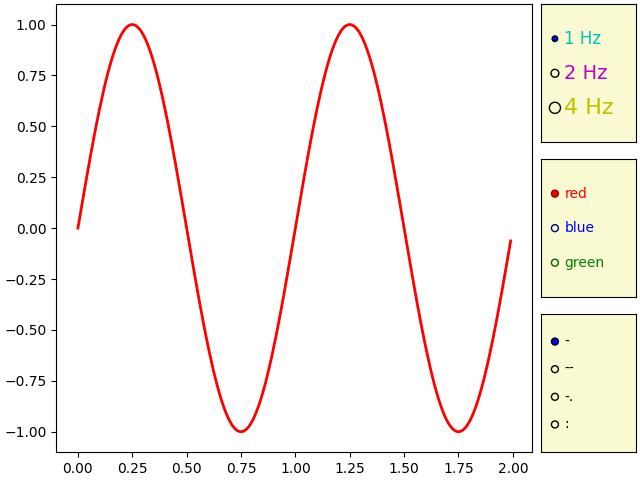
脚本总运行时间:(0 分钟 1.268 秒)