注意
转到末尾 下载完整示例代码。
受限布局指南#
使用受限布局将绘图整齐地拟合到您的图形中。
受限布局会自动调整子图,以便刻度标签、图例和颜色条等装饰不会重叠,同时仍然保留用户请求的逻辑布局。
受约束布局类似于紧凑布局,但更加灵活。它可以处理放置在多个坐标轴上的颜色条 (放置颜色条) 嵌套布局 (subfigures
) 以及跨行或跨列的坐标轴 (subplot_mosaic
),努力对齐同一行或同一列中坐标轴的脊柱。此外,压缩布局 将尝试将固定纵横比的坐标轴移到更靠近的位置。这些功能将在本文档中进行描述,以及最后讨论的一些实现细节。
受约束布局通常需要在将任何坐标轴添加到图形之前激活。有两种方法可以做到这一点
使用
subplots
、figure
、subplot_mosaic
的相应参数,例如plt.subplots(layout="constrained")
通过rcParams 激活它,例如
plt.rcParams['figure.constrained_layout.use'] = True
这些将在以下各节中详细描述。
警告
调用tight_layout
将关闭受约束布局!
简单示例#
使用默认的坐标轴定位,坐标轴标题、轴标签或刻度标签有时会超出图形区域,从而被裁剪。
import matplotlib.pyplot as plt
import numpy as np
import matplotlib.colors as mcolors
import matplotlib.gridspec as gridspec
plt.rcParams['savefig.facecolor'] = "0.8"
plt.rcParams['figure.figsize'] = 4.5, 4.
plt.rcParams['figure.max_open_warning'] = 50
def example_plot(ax, fontsize=12, hide_labels=False):
ax.plot([1, 2])
ax.locator_params(nbins=3)
if hide_labels:
ax.set_xticklabels([])
ax.set_yticklabels([])
else:
ax.set_xlabel('x-label', fontsize=fontsize)
ax.set_ylabel('y-label', fontsize=fontsize)
ax.set_title('Title', fontsize=fontsize)
fig, ax = plt.subplots(layout=None)
example_plot(ax, fontsize=24)
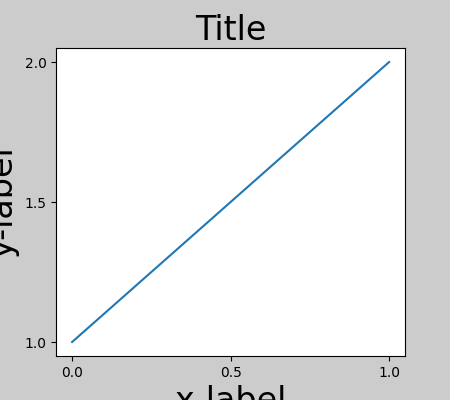
为了避免这种情况,需要调整 Axes 的位置。对于子图,可以通过使用 Figure.subplots_adjust
手动调整子图参数来实现。但是,使用 layout="constrained"
关键字参数指定图形会自动进行调整。
fig, ax = plt.subplots(layout="constrained")
example_plot(ax, fontsize=24)
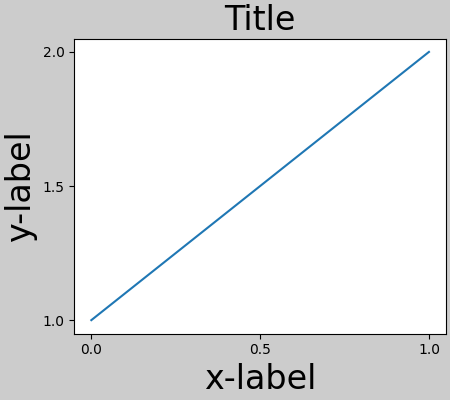
当有多个子图时,经常会看到不同 Axes 的标签互相重叠。
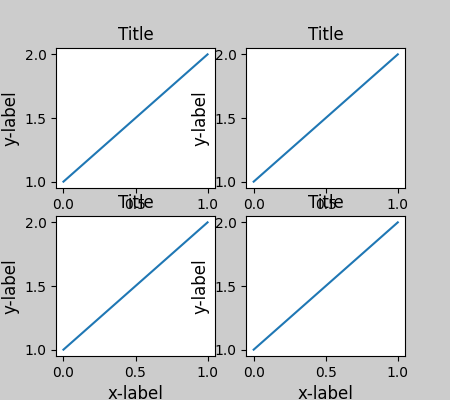
在调用 plt.subplots
时指定 layout="constrained"
会使布局得到适当的约束。
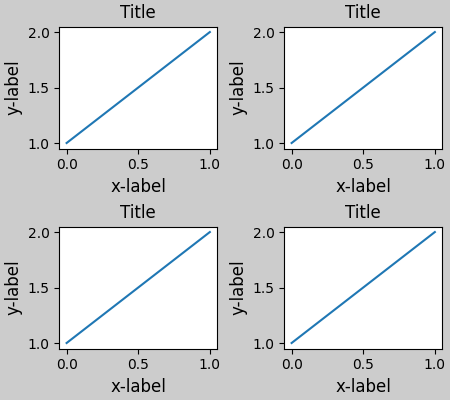
颜色条#
如果使用 Figure.colorbar
创建颜色条,则需要为其留出空间。约束布局 会自动完成此操作。请注意,如果指定 use_gridspec=True
,它将被忽略,因为此选项是为了通过 tight_layout
改善布局而设计的。
注意
对于 pcolormesh
关键字参数 (pc_kwargs
),我们使用字典来保持整个文档中调用的一致性。
arr = np.arange(100).reshape((10, 10))
norm = mcolors.Normalize(vmin=0., vmax=100.)
# see note above: this makes all pcolormesh calls consistent:
pc_kwargs = {'rasterized': True, 'cmap': 'viridis', 'norm': norm}
fig, ax = plt.subplots(figsize=(4, 4), layout="constrained")
im = ax.pcolormesh(arr, **pc_kwargs)
fig.colorbar(im, ax=ax, shrink=0.6)
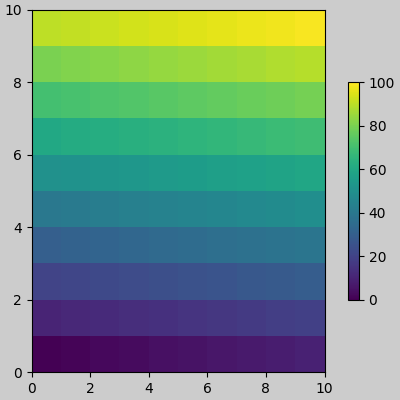
如果将 Axes 列表(或其他可迭代容器)指定给 colorbar
的 ax
参数,约束布局 将从指定的 Axes 中获取空间。
fig, axs = plt.subplots(2, 2, figsize=(4, 4), layout="constrained")
for ax in axs.flat:
im = ax.pcolormesh(arr, **pc_kwargs)
fig.colorbar(im, ax=axs, shrink=0.6)
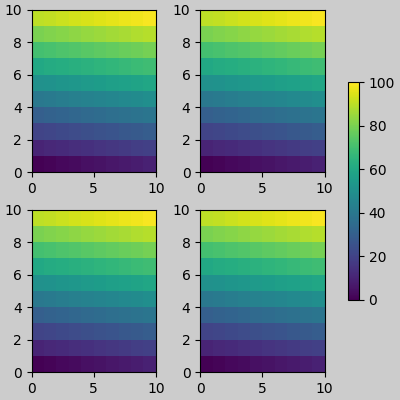
如果从 Axes 网格中指定 Axes 列表,颜色条将适当地占用空间并留出间隙,但所有子图的大小仍然相同。
fig, axs = plt.subplots(3, 3, figsize=(4, 4), layout="constrained")
for ax in axs.flat:
im = ax.pcolormesh(arr, **pc_kwargs)
fig.colorbar(im, ax=axs[1:, 1], shrink=0.8)
fig.colorbar(im, ax=axs[:, -1], shrink=0.6)
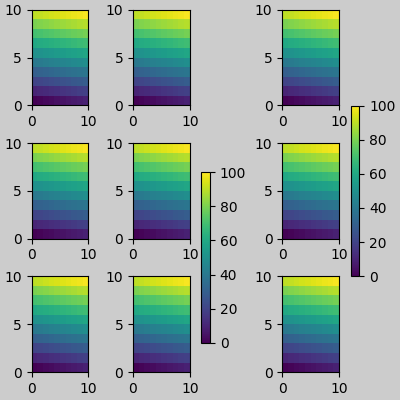
总标题#
受限布局 也可以为 suptitle
预留空间。
fig, axs = plt.subplots(2, 2, figsize=(4, 4), layout="constrained")
for ax in axs.flat:
im = ax.pcolormesh(arr, **pc_kwargs)
fig.colorbar(im, ax=axs, shrink=0.6)
fig.suptitle('Big Suptitle')
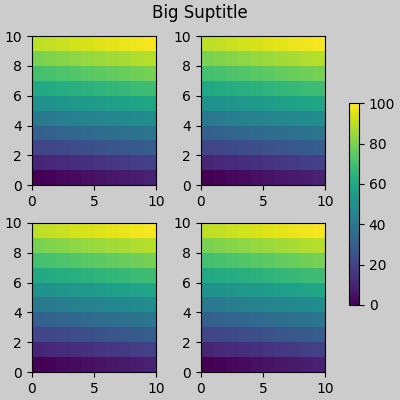
图例#
图例可以放置在其父轴之外。受限布局 旨在为 Axes.legend()
处理这种情况。但是,受限布局 不 处理通过 Figure.legend()
创建的图例(尚未)。
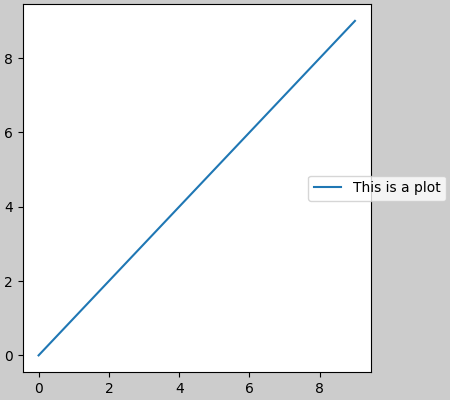
但是,这将从子图布局中窃取空间
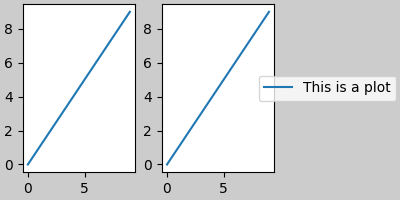
为了使图例或其他艺术家不 从子图布局中窃取空间,我们可以 leg.set_in_layout(False)
。当然,这意味着图例最终会被裁剪,但如果随后使用 fig.savefig('outname.png', bbox_inches='tight')
调用绘图,这将很有用。但是请注意,图例的 get_in_layout
状态将必须再次切换才能使保存的文件正常工作,并且如果我们希望受限布局 在打印之前调整 Axes 的大小,我们必须手动触发绘制。
fig, axs = plt.subplots(1, 2, figsize=(4, 2), layout="constrained")
axs[0].plot(np.arange(10))
axs[1].plot(np.arange(10), label='This is a plot')
leg = axs[1].legend(loc='center left', bbox_to_anchor=(0.8, 0.5))
leg.set_in_layout(False)
# trigger a draw so that constrained layout is executed once
# before we turn it off when printing....
fig.canvas.draw()
# we want the legend included in the bbox_inches='tight' calcs.
leg.set_in_layout(True)
# we don't want the layout to change at this point.
fig.set_layout_engine('none')
try:
fig.savefig('../../../doc/_static/constrained_layout_1b.png',
bbox_inches='tight', dpi=100)
except FileNotFoundError:
# this allows the script to keep going if run interactively and
# the directory above doesn't exist
pass
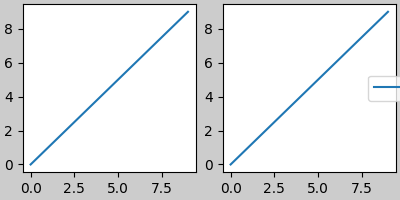
保存的文件看起来像
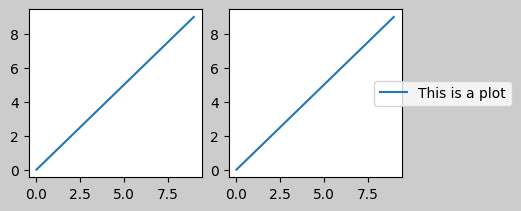
解决这种尴尬的更好方法是简单地使用 Figure.legend
提供的图例方法
fig, axs = plt.subplots(1, 2, figsize=(4, 2), layout="constrained")
axs[0].plot(np.arange(10))
lines = axs[1].plot(np.arange(10), label='This is a plot')
labels = [l.get_label() for l in lines]
leg = fig.legend(lines, labels, loc='center left',
bbox_to_anchor=(0.8, 0.5), bbox_transform=axs[1].transAxes)
try:
fig.savefig('../../../doc/_static/constrained_layout_2b.png',
bbox_inches='tight', dpi=100)
except FileNotFoundError:
# this allows the script to keep going if run interactively and
# the directory above doesn't exist
pass
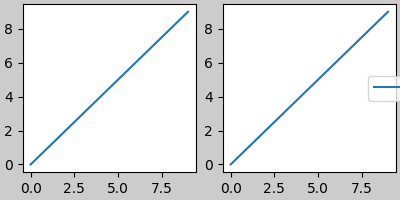
保存的文件看起来像
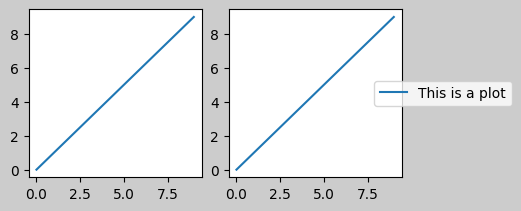
填充和间距#
轴之间的填充在水平方向上由w_pad 和wspace 控制,在垂直方向上由h_pad 和hspace 控制。这些可以通过 set
编辑。w/h_pad 是轴周围的最小空间,以英寸为单位
fig, axs = plt.subplots(2, 2, layout="constrained")
for ax in axs.flat:
example_plot(ax, hide_labels=True)
fig.get_layout_engine().set(w_pad=4 / 72, h_pad=4 / 72, hspace=0,
wspace=0)
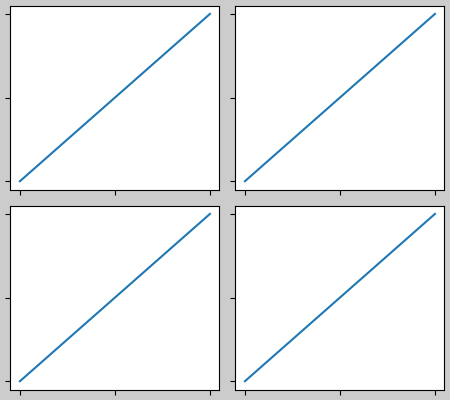
子图之间的间距由wspace 和hspace 进一步设置。这些指定为整个子图组大小的一部分。如果这些值小于w_pad 或h_pad,则使用固定填充。请注意,在下面,边缘的空间没有从上面改变,但子图之间的空间确实改变了。
fig, axs = plt.subplots(2, 2, layout="constrained")
for ax in axs.flat:
example_plot(ax, hide_labels=True)
fig.get_layout_engine().set(w_pad=4 / 72, h_pad=4 / 72, hspace=0.2,
wspace=0.2)
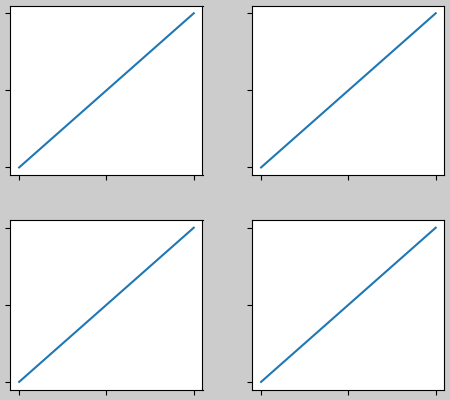
如果有多于两列,则wspace在它们之间共享,因此此处wspace被分成两部分,每列之间wspace为0.1。
fig, axs = plt.subplots(2, 3, layout="constrained")
for ax in axs.flat:
example_plot(ax, hide_labels=True)
fig.get_layout_engine().set(w_pad=4 / 72, h_pad=4 / 72, hspace=0.2,
wspace=0.2)
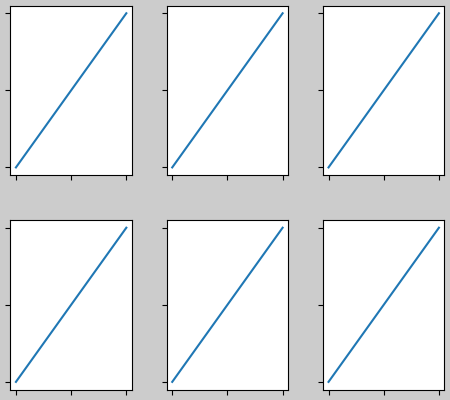
GridSpecs 也有可选的hspace 和 wspace 关键字参数,它们将用于代替由受限布局设置的填充。
fig, axs = plt.subplots(2, 2, layout="constrained",
gridspec_kw={'wspace': 0.3, 'hspace': 0.2})
for ax in axs.flat:
example_plot(ax, hide_labels=True)
# this has no effect because the space set in the gridspec trumps the
# space set in *constrained layout*.
fig.get_layout_engine().set(w_pad=4 / 72, h_pad=4 / 72, hspace=0.0,
wspace=0.0)
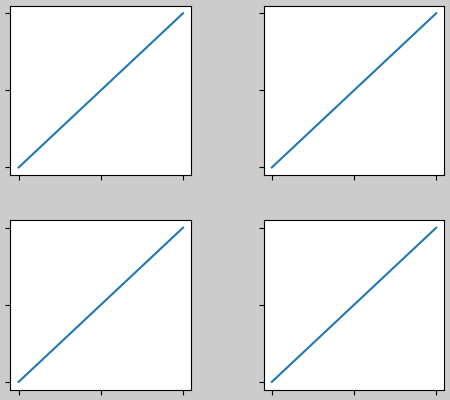
带颜色条的间距#
颜色条与其父级之间保持pad距离,其中pad是父级宽度的一部分。然后,与下一个子图的间距由w/hspace给出。
fig, axs = plt.subplots(2, 2, layout="constrained")
pads = [0, 0.05, 0.1, 0.2]
for pad, ax in zip(pads, axs.flat):
pc = ax.pcolormesh(arr, **pc_kwargs)
fig.colorbar(pc, ax=ax, shrink=0.6, pad=pad)
ax.set_xticklabels([])
ax.set_yticklabels([])
ax.set_title(f'pad: {pad}')
fig.get_layout_engine().set(w_pad=2 / 72, h_pad=2 / 72, hspace=0.2,
wspace=0.2)
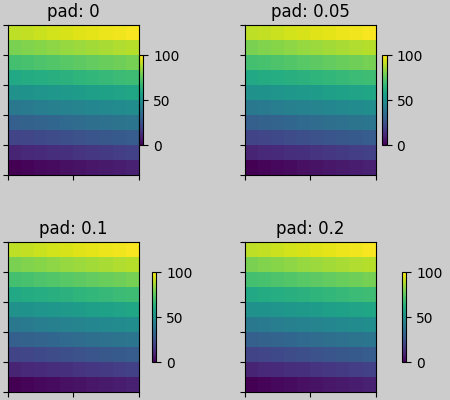
rcParams#
有五个rcParams可以设置,无论是在脚本中还是在matplotlibrc
文件中。它们都以figure.constrained_layout
为前缀。
use: 是否使用受限布局。默认值为 False。
w_pad, h_pad: 围绕 Axes 对象的填充。表示英寸的浮点数。默认值为 3./72. 英寸(3 点)。
wspace, hspace: 子图组之间的间距。表示子图宽度分数的浮点数。默认值为 0.02。
plt.rcParams['figure.constrained_layout.use'] = True
fig, axs = plt.subplots(2, 2, figsize=(3, 3))
for ax in axs.flat:
example_plot(ax)
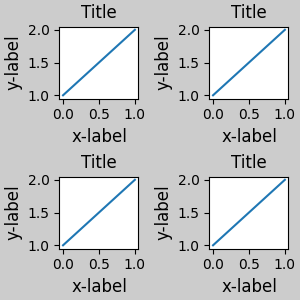
与 GridSpec 一起使用#
受限布局旨在与subplots()
、subplot_mosaic()
或 GridSpec()
以及 add_subplot()
一起使用。
请注意,在以下内容中,layout="constrained"
plt.rcParams['figure.constrained_layout.use'] = False
fig = plt.figure(layout="constrained")
gs1 = gridspec.GridSpec(2, 1, figure=fig)
ax1 = fig.add_subplot(gs1[0])
ax2 = fig.add_subplot(gs1[1])
example_plot(ax1)
example_plot(ax2)
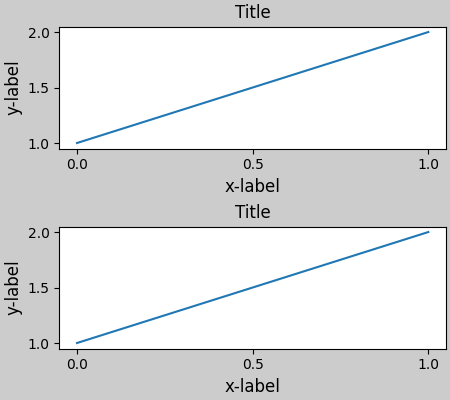
更复杂的 gridspec 布局是可能的。请注意,这里我们使用便利函数 add_gridspec
和 subgridspec
。
fig = plt.figure(layout="constrained")
gs0 = fig.add_gridspec(1, 2)
gs1 = gs0[0].subgridspec(2, 1)
ax1 = fig.add_subplot(gs1[0])
ax2 = fig.add_subplot(gs1[1])
example_plot(ax1)
example_plot(ax2)
gs2 = gs0[1].subgridspec(3, 1)
for ss in gs2:
ax = fig.add_subplot(ss)
example_plot(ax)
ax.set_title("")
ax.set_xlabel("")
ax.set_xlabel("x-label", fontsize=12)
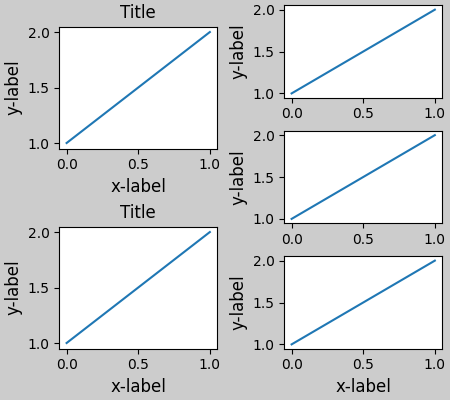
请注意,在上面,左右两列的垂直范围不同。如果我们希望两个网格的顶部和底部对齐,那么它们需要在同一个 gridspec 中。为了防止 Axes 塌缩为零高度,我们还需要将此图形放大。
fig = plt.figure(figsize=(4, 6), layout="constrained")
gs0 = fig.add_gridspec(6, 2)
ax1 = fig.add_subplot(gs0[:3, 0])
ax2 = fig.add_subplot(gs0[3:, 0])
example_plot(ax1)
example_plot(ax2)
ax = fig.add_subplot(gs0[0:2, 1])
example_plot(ax, hide_labels=True)
ax = fig.add_subplot(gs0[2:4, 1])
example_plot(ax, hide_labels=True)
ax = fig.add_subplot(gs0[4:, 1])
example_plot(ax, hide_labels=True)
fig.suptitle('Overlapping Gridspecs')
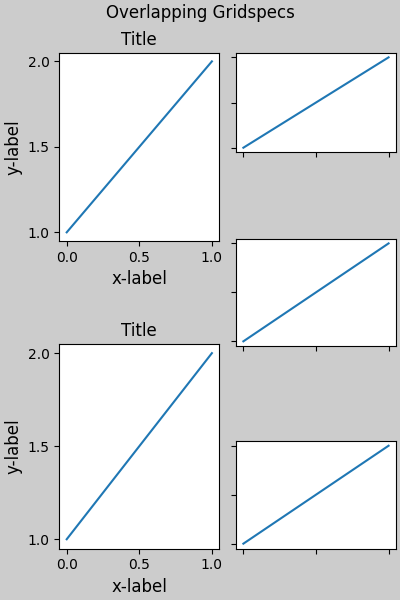
此示例使用两个网格规格,使颜色条仅与一组 pcolor 相关联。注意,由于此原因,左列比右边的两列更宽。当然,如果您希望子图大小相同,您只需要一个网格规格。请注意,可以使用 subfigures
达到相同的效果。
fig = plt.figure(layout="constrained")
gs0 = fig.add_gridspec(1, 2, figure=fig, width_ratios=[1, 2])
gs_left = gs0[0].subgridspec(2, 1)
gs_right = gs0[1].subgridspec(2, 2)
for gs in gs_left:
ax = fig.add_subplot(gs)
example_plot(ax)
axs = []
for gs in gs_right:
ax = fig.add_subplot(gs)
pcm = ax.pcolormesh(arr, **pc_kwargs)
ax.set_xlabel('x-label')
ax.set_ylabel('y-label')
ax.set_title('title')
axs += [ax]
fig.suptitle('Nested plots using subgridspec')
fig.colorbar(pcm, ax=axs)
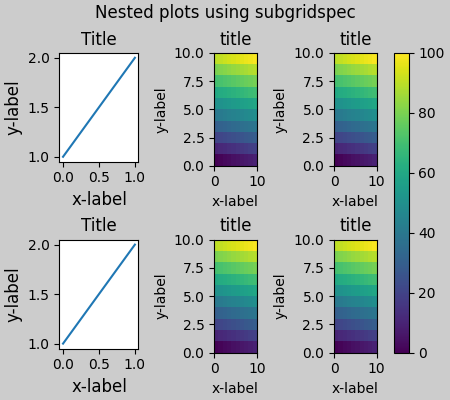
Matplotlib 现在提供了 subfigures
,它也适用于 *受约束的布局*,而不是使用子网格规格。
fig = plt.figure(layout="constrained")
sfigs = fig.subfigures(1, 2, width_ratios=[1, 2])
axs_left = sfigs[0].subplots(2, 1)
for ax in axs_left.flat:
example_plot(ax)
axs_right = sfigs[1].subplots(2, 2)
for ax in axs_right.flat:
pcm = ax.pcolormesh(arr, **pc_kwargs)
ax.set_xlabel('x-label')
ax.set_ylabel('y-label')
ax.set_title('title')
fig.colorbar(pcm, ax=axs_right)
fig.suptitle('Nested plots using subfigures')
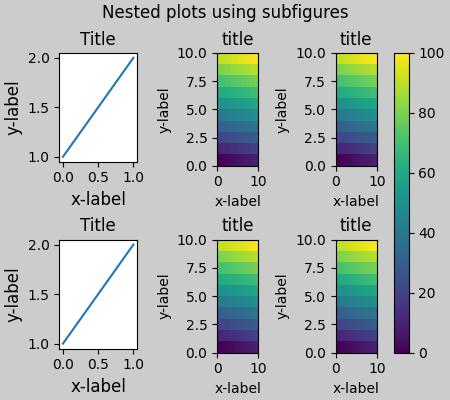
手动设置 Axes 位置#
手动设置 Axes 位置可能会有很好的理由。对 set_position
的手动调用将设置 Axes,因此 *受约束的布局* 对它不再有任何影响。(请注意,*受约束的布局* 仍然保留移动的 Axes 的空间)。
fig, axs = plt.subplots(1, 2, layout="constrained")
example_plot(axs[0], fontsize=12)
axs[1].set_position([0.2, 0.2, 0.4, 0.4])
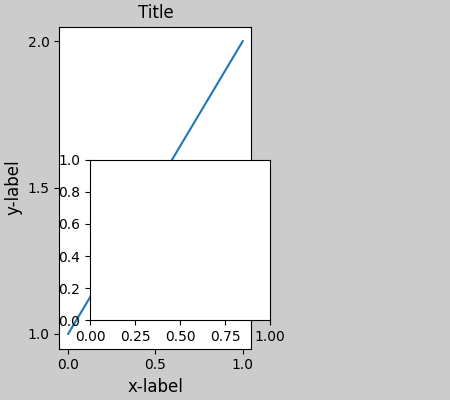
固定纵横比 Axes 的网格:“压缩”布局#
*受约束的布局* 在 Axes 的“原始”位置网格上运行。但是,当 Axes 具有固定纵横比时,通常会使一侧变短,并在缩短的方向上留下很大的间隙。在下面,Axes 是正方形,但图形很宽,因此存在水平间隙
fig, axs = plt.subplots(2, 2, figsize=(5, 3),
sharex=True, sharey=True, layout="constrained")
for ax in axs.flat:
ax.imshow(arr)
fig.suptitle("fixed-aspect plots, layout='constrained'")
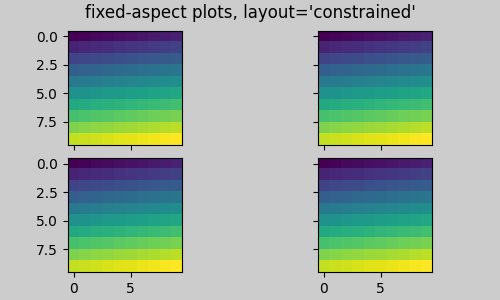
解决此问题的一种明显方法是使图形大小更接近正方形,但是,精确地关闭间隙需要反复试验。对于 Axes 的简单网格,我们可以使用 layout="compressed"
为我们完成这项工作
fig, axs = plt.subplots(2, 2, figsize=(5, 3),
sharex=True, sharey=True, layout='compressed')
for ax in axs.flat:
ax.imshow(arr)
fig.suptitle("fixed-aspect plots, layout='compressed'")
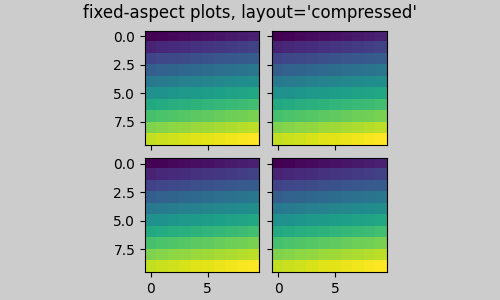
手动关闭 *受约束的布局*#
*受约束的布局* 通常在图形的每次绘制时调整 Axes 位置。如果您想获得 *受约束的布局* 提供的间距,但不想让它更新,那么请进行初始绘制,然后调用 fig.set_layout_engine('none')
。这对于刻度标签长度可能发生变化的动画可能很有用。
请注意,对于使用工具栏的后端,*受约束的布局* 在 ZOOM
和 PAN
GUI 事件中被关闭。这可以防止 Axes 在缩放和平移期间改变位置。
局限性#
不兼容的功能#
受限布局 将与 pyplot.subplot
一起工作,但前提是每次调用的行数和列数必须相同。原因是,如果几何形状不同,每次调用 pyplot.subplot
将创建一个新的 GridSpec
实例,而受限布局。因此,以下代码可以正常工作
fig = plt.figure(layout="constrained")
ax1 = plt.subplot(2, 2, 1)
ax2 = plt.subplot(2, 2, 3)
# third Axes that spans both rows in second column:
ax3 = plt.subplot(2, 2, (2, 4))
example_plot(ax1)
example_plot(ax2)
example_plot(ax3)
plt.suptitle('Homogenous nrows, ncols')
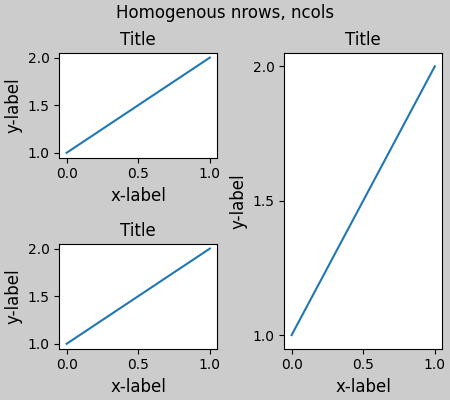
但以下代码会导致布局不佳
fig = plt.figure(layout="constrained")
ax1 = plt.subplot(2, 2, 1)
ax2 = plt.subplot(2, 2, 3)
ax3 = plt.subplot(1, 2, 2)
example_plot(ax1)
example_plot(ax2)
example_plot(ax3)
plt.suptitle('Mixed nrows, ncols')
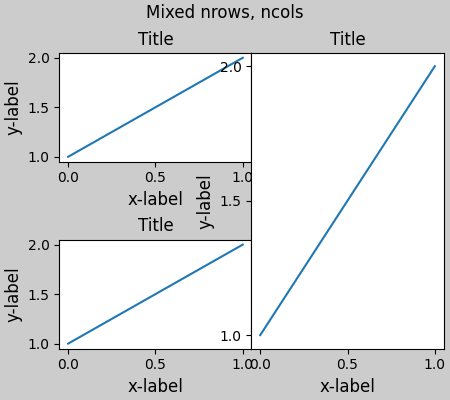
类似地,subplot2grid
也存在相同的限制,即 nrows 和 ncols 必须保持不变,才能使布局看起来良好。
fig = plt.figure(layout="constrained")
ax1 = plt.subplot2grid((3, 3), (0, 0))
ax2 = plt.subplot2grid((3, 3), (0, 1), colspan=2)
ax3 = plt.subplot2grid((3, 3), (1, 0), colspan=2, rowspan=2)
ax4 = plt.subplot2grid((3, 3), (1, 2), rowspan=2)
example_plot(ax1)
example_plot(ax2)
example_plot(ax3)
example_plot(ax4)
fig.suptitle('subplot2grid')
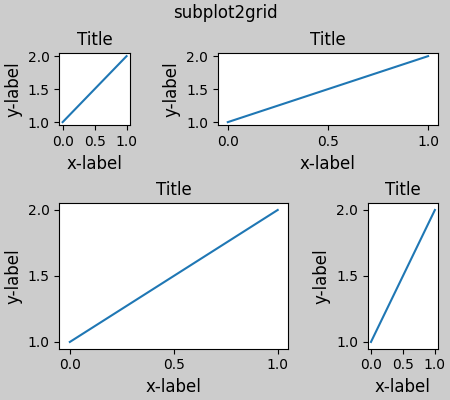
其他注意事项#
受限布局 仅考虑刻度标签、轴标签、标题和图例。因此,其他绘图元素可能会被裁剪,也可能重叠。
它假设刻度标签、轴标签和标题所需的额外空间与 Axes 的原始位置无关。这通常是正确的,但也有少数情况并非如此。
后端处理渲染字体的机制略有不同,因此结果不会完全相同。
如果艺术家使用超出 Axes 边界的 Axes 坐标,则添加到 Axes 时会导致布局异常。可以通过使用
Figure
的add_artist()
将艺术家直接添加到Figure
来避免这种情况。有关示例,请参见ConnectionPatch
。
调试#
受限布局可能会以一些意想不到的方式失败。因为它使用约束求解器,所以求解器可以找到数学上正确的解,但这些解根本不是用户想要的。通常的失败模式是所有大小都缩小到其允许的最小值。如果发生这种情况,则可能是以下两个原因之一
您请求绘制的元素没有足够的空间。
存在错误 - 在这种情况下,请在 matplotlib/matplotlib#issues 上打开一个问题。
如果存在错误,请报告一个自包含的示例,该示例不需要外部数据或依赖项(除了 numpy)。
关于算法的说明#
约束算法相对简单,但由于我们以复杂的方式布局图形,因此具有一定的复杂性。
Matplotlib 中的布局通过 GridSpec
类使用网格规范来执行。网格规范是将图形逻辑地划分为行和列,其中这些行和列中 Axes 的相对宽度由 width_ratios 和 height_ratios 设置。
在受限布局中,每个网格规格都关联一个布局网格。布局网格为每一列有一系列的left
和right
变量,为每一行有一系列的bottom
和top
变量,此外它还为左、右、下和上设置了边距。在每一行中,下/上边距会一直扩大,直到容纳该行中的所有装饰器。类似地,对于列和左/右边距也是如此。
简单案例:一个 Axes#
对于单个 Axes,布局非常简单。图形有一个包含一列一行的父布局网格,以及一个包含 Axes 的网格规格的子布局网格,同样包含一行一列。为 Axes 两侧的“装饰”留出空间。在代码中,这是通过do_constrained_layout()
中的条目实现的,例如
gridspec._layoutgrid[0, 0].edit_margin_min('left',
-bbox.x0 + pos.x0 + w_pad)
其中bbox
是 Axes 的紧密边界框,pos
是其位置。请注意,四个边距是如何包含 Axes 装饰的。
from matplotlib._layoutgrid import plot_children
fig, ax = plt.subplots(layout="constrained")
example_plot(ax, fontsize=24)
plot_children(fig)
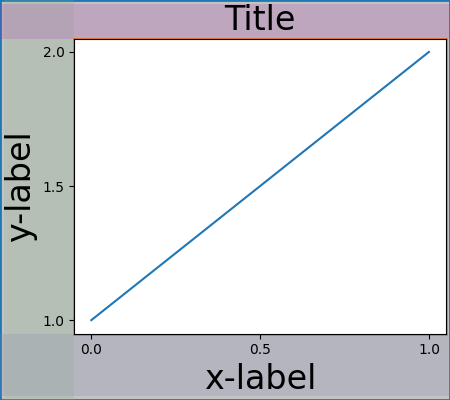
简单案例:两个 Axes#
当有多个 Axes 时,它们的布局以简单的方式绑定在一起。在这个例子中,左侧 Axes 的装饰比右侧的要大得多,但它们共享一个底部边距,该边距被放大到足以容纳更大的 xlabel。共享的顶部边距也是如此。左右边距不共享,因此允许它们不同。
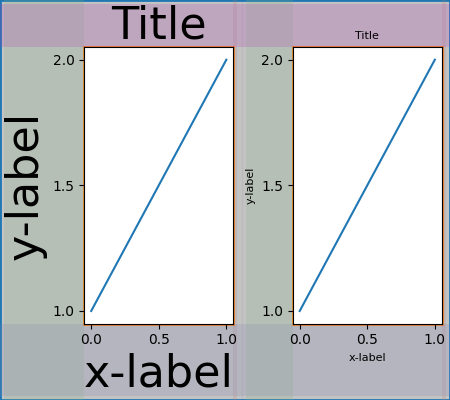
两个 Axes 和颜色条#
颜色条只是另一个扩展父布局网格单元边距的项目
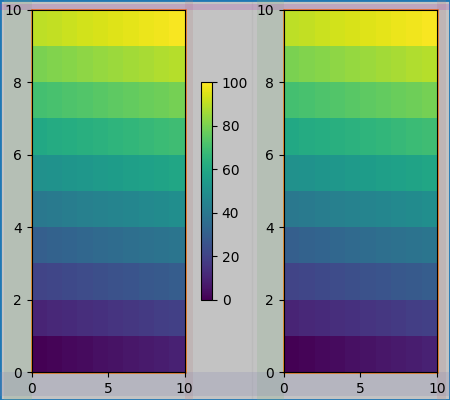
与网格规格关联的颜色条#
如果颜色条属于网格的多个单元格,那么它会为每个单元格设置更大的边距
fig, axs = plt.subplots(2, 2, layout="constrained")
for ax in axs.flat:
im = ax.pcolormesh(arr, **pc_kwargs)
fig.colorbar(im, ax=axs, shrink=0.6)
plot_children(fig)
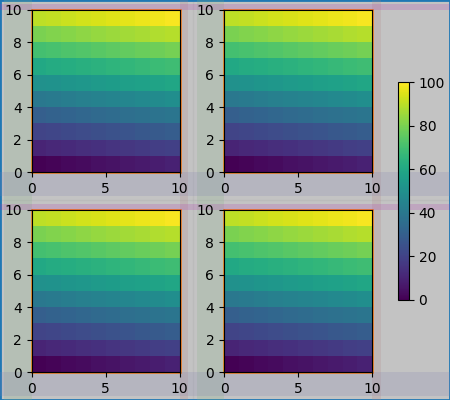
大小不一的 Axes#
有两种方法可以使 Axes 在网格规格布局中具有不均匀的大小,要么指定它们跨越网格规格的行或列,要么指定宽度和高度比率。
这里使用第一种方法。请注意,中间的top
和bottom
边距不受左侧列的影响。这是算法的刻意决定,导致两个右侧 Axes 具有相同的高度,但它不是左侧 Axes 高度的一半。这与gridspec
在没有受限布局的情况下工作方式一致。
fig = plt.figure(layout="constrained")
gs = gridspec.GridSpec(2, 2, figure=fig)
ax = fig.add_subplot(gs[:, 0])
im = ax.pcolormesh(arr, **pc_kwargs)
ax = fig.add_subplot(gs[0, 1])
im = ax.pcolormesh(arr, **pc_kwargs)
ax = fig.add_subplot(gs[1, 1])
im = ax.pcolormesh(arr, **pc_kwargs)
plot_children(fig)
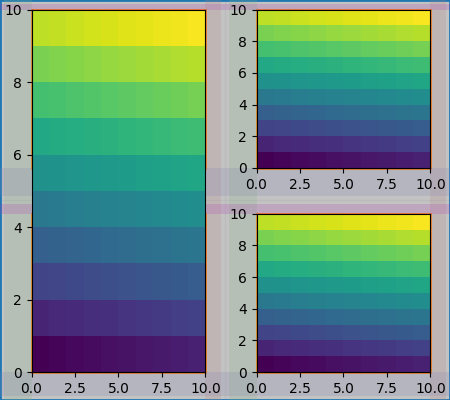
需要调整的一个情况是,如果边距没有任何艺术家来约束它们的宽度。在下面的情况下,第 0 列的右边距和第 3 列的左边距没有边距艺术家来设置它们的宽度,因此我们取具有艺术家的边距宽度的最大宽度。这使得所有 Axes 具有相同的大小
fig = plt.figure(layout="constrained")
gs = fig.add_gridspec(2, 4)
ax00 = fig.add_subplot(gs[0, 0:2])
ax01 = fig.add_subplot(gs[0, 2:])
ax10 = fig.add_subplot(gs[1, 1:3])
example_plot(ax10, fontsize=14)
plot_children(fig)
plt.show()
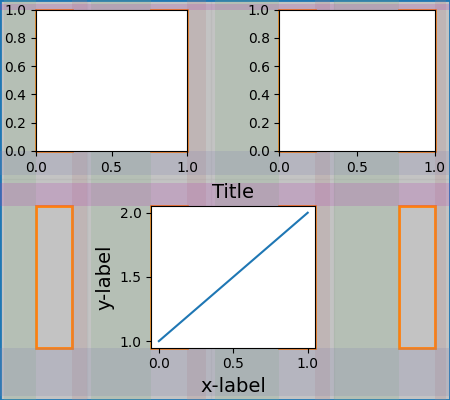
脚本总运行时间: (0 分钟 21.737 秒)