注意
跳转到末尾 下载完整的示例代码。
艺术家教程#
使用 Artist 对象在画布上渲染。
Matplotlib API 有三个层次。
matplotlib.backend_bases.FigureCanvas
是绘制图形的区域matplotlib.backend_bases.Renderer
是知道如何在matplotlib.backend_bases.FigureCanvas
上绘制的对象并且
matplotlib.artist.Artist
是知道如何使用渲染器在画布上绘制的对象。
matplotlib.backend_bases.FigureCanvas
和 matplotlib.backend_bases.Renderer
处理与用户界面工具包(如 wxPython)或绘图语言(如 PostScript®)进行交互的所有细节,而 Artist
处理所有高级结构,如表示和布局图形、文本和线条。典型用户 95% 的时间将用于使用 Artists
。
有两类 Artists
:基本元素和容器。基本元素代表我们要绘制到画布上的标准图形对象:Line2D
、Rectangle
、Text
、AxesImage
等,而容器是放置它们的地方(Axis
、Axes
和 Figure
)。标准用法是创建 Figure
实例,使用 Figure
创建一个或多个 Axes
实例,并使用 Axes
实例辅助方法创建基本元素。在下面的示例中,我们使用 matplotlib.pyplot.figure()
创建 Figure
实例,这是一个实例化 Figure
实例并将其与用户界面或绘图工具包 FigureCanvas
连接的便捷方法。正如我们将在下面讨论的,这不是必需的——您可以直接使用 PostScript、PDF Gtk+ 或 wxPython FigureCanvas
实例,直接实例化您的 Figures
并自行连接它们——但由于我们在这里专注于 Artist
API,我们将让 pyplot
为我们处理其中的一些细节
import matplotlib.pyplot as plt
fig = plt.figure()
ax = fig.add_subplot(2, 1, 1) # two rows, one column, first plot
Axes
可能是 Matplotlib API 中最重要的类,也是您大部分时间将要使用的类。这是因为 Axes
是大多数对象进入的绘图区域,并且 Axes
有许多特殊的辅助方法(plot()
、text()
、hist()
、imshow()
)来创建最常见的图形基本元素(分别是 Line2D
、Text
、Rectangle
、AxesImage
)。这些辅助方法将采用您的数据(例如,numpy
数组和字符串)并根据需要创建基本 Artist
实例(例如,Line2D
),将它们添加到相关的容器,并在请求时绘制它们。如果要以任意位置创建 Axes
,只需使用 add_axes()
方法,该方法采用 0-1 相对图形坐标中的 [left, bottom, width, height]
值列表
fig2 = plt.figure()
ax2 = fig2.add_axes([0.15, 0.1, 0.7, 0.3])
继续我们的示例
在此示例中,ax
是由上面的 fig.add_subplot
调用创建的 Axes
实例,当您调用 ax.plot
时,它会创建一个 Line2D
实例并将其添加到 Axes
。在下面的交互式 IPython 会话中,您可以看到 Axes.lines
列表的长度为 1,并且包含与 line, = ax.plot...
调用返回的同一条线
如果您后续调用 ax.plot
(并且保持状态为“on”,这是默认状态),则其他线条将添加到列表中。您可以稍后通过调用其 remove
方法来删除一条线
Axes 还具有辅助方法来配置和装饰 x 轴和 y 轴刻度、刻度标签和轴标签
当您调用 ax.set_xlabel
时,它会将信息传递给 Text
实例 XAxis
。每个 Axes
实例都包含一个 XAxis
和一个 YAxis
实例,它们处理刻度、刻度标签和轴标签的布局和绘制。
尝试创建下图。
import matplotlib.pyplot as plt
import numpy as np
fig = plt.figure()
fig.subplots_adjust(top=0.8)
ax1 = fig.add_subplot(211)
ax1.set_ylabel('Voltage [V]')
ax1.set_title('A sine wave')
t = np.arange(0.0, 1.0, 0.01)
s = np.sin(2*np.pi*t)
line, = ax1.plot(t, s, color='blue', lw=2)
# Fixing random state for reproducibility
np.random.seed(19680801)
ax2 = fig.add_axes([0.15, 0.1, 0.7, 0.3])
n, bins, patches = ax2.hist(np.random.randn(1000), 50,
facecolor='yellow', edgecolor='yellow')
ax2.set_xlabel('Time [s]')
plt.show()
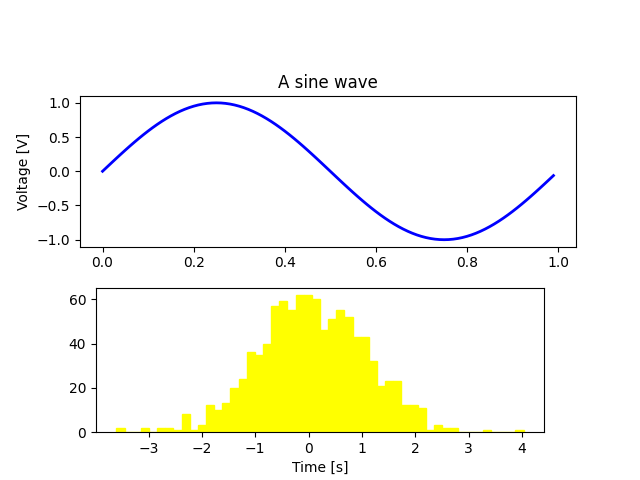
自定义对象#
图形中的每个元素都由 Matplotlib Artist
表示,每个都有一个广泛的属性列表来配置其外观。图形本身包含一个大小与图形完全相同的 Rectangle
,您可以使用它来设置图形的背景颜色和透明度。同样,每个 Axes
边界框(典型 Matplotlib 绘图中带有黑色边缘的标准白色框)都有一个 Rectangle
实例,该实例确定 Axes 的颜色、透明度和其他属性。这些实例存储为成员变量 Figure.patch
和 Axes.patch
(“Patch”是继承自 MATLAB 的名称,是图形上 2D 颜色的“补丁”,例如矩形、圆形和多边形)。每个 Matplotlib Artist
都有以下属性
属性 |
描述 |
---|---|
alpha |
透明度 - 从 0 到 1 的标量 |
animated |
一个布尔值,用于促进动画绘制 |
axes |
Artist 所在的 Axes,可能为 None |
clip_box |
裁剪 Artist 的边界框 |
clip_on |
是否启用裁剪 |
clip_path |
艺术家被裁剪到的路径 |
contains |
用于测试艺术家是否包含拾取点的拾取函数 |
figure |
艺术家所在的 figure 实例,可能为 None |
label |
文本标签(例如,用于自动标签) |
picker |
控制对象拾取的 Python 对象 |
transform |
变换 |
visible |
布尔值,指示是否应绘制艺术家 |
zorder |
确定绘制顺序的数字 |
rasterized |
布尔值;将矢量图形转换为栅格图形(用于压缩和 EPS 透明度) |
每个属性都使用老式的 setter 或 getter 进行访问(是的,我们知道这会激怒 Pythonista,我们计划支持通过属性或特征直接访问,但这尚未完成)。例如,要将当前 alpha 乘以一半
a = o.get_alpha()
o.set_alpha(0.5*a)
如果想一次设置多个属性,也可以使用带有关键字参数的 set
方法。例如
o.set(alpha=0.5, zorder=2)
如果您在 Python shell 中以交互方式工作,一种方便的检查 Artist
属性的方法是使用 matplotlib.artist.getp()
函数(在 pyplot 中简称为 getp()
),它列出了属性及其值。这对于从 Artist
派生的类也适用,例如 Figure
和 Rectangle
。以下是上面提到的 Figure
矩形属性
In [149]: matplotlib.artist.getp(fig.patch)
agg_filter = None
alpha = None
animated = False
antialiased or aa = False
bbox = Bbox(x0=0.0, y0=0.0, x1=1.0, y1=1.0)
capstyle = butt
children = []
clip_box = None
clip_on = True
clip_path = None
contains = None
data_transform = BboxTransformTo( TransformedBbox( Bbox...
edgecolor or ec = (1.0, 1.0, 1.0, 1.0)
extents = Bbox(x0=0.0, y0=0.0, x1=640.0, y1=480.0)
facecolor or fc = (1.0, 1.0, 1.0, 1.0)
figure = Figure(640x480)
fill = True
gid = None
hatch = None
height = 1
in_layout = False
joinstyle = miter
label =
linestyle or ls = solid
linewidth or lw = 0.0
patch_transform = CompositeGenericTransform( BboxTransformTo( ...
path = Path(array([[0., 0.], [1., 0.], [1.,...
path_effects = []
picker = None
rasterized = None
sketch_params = None
snap = None
transform = CompositeGenericTransform( CompositeGenericTra...
transformed_clip_path_and_affine = (None, None)
url = None
verts = [[ 0. 0.] [640. 0.] [640. 480.] [ 0. 480....
visible = True
width = 1
window_extent = Bbox(x0=0.0, y0=0.0, x1=640.0, y1=480.0)
x = 0
xy = (0, 0)
y = 0
zorder = 1
所有类的文档字符串也包含 Artist
属性,因此您可以查阅交互式“帮助”或 matplotlib.artist 以获取给定对象的属性列表。
对象容器#
现在我们知道如何检查和设置要配置的给定对象的属性,我们需要知道如何访问该对象。如引言中所述,有两种类型的对象:基本对象和容器。基本对象通常是您要配置的内容(Text
实例的字体,Line2D
的宽度),尽管容器也有一些属性,例如,Axes
Artist
是一个容器,其中包含绘图中许多基本对象,但它也具有诸如 xscale
之类的属性,用于控制 x 轴是“线性”还是“对数”。在本节中,我们将回顾各种容器对象在其中存储您要访问的 Artists
。
Figure 容器#
顶层容器 Artist
是 matplotlib.figure.Figure
,它包含图形中的所有内容。图形的背景是 Rectangle
,存储在 Figure.patch
中。当您向图形添加子图(add_subplot()
)和 Axes(add_axes()
)时,它们将被附加到 Figure.axes
。这些方法也会返回创建的子图和 Axes
In [156]: fig = plt.figure()
In [157]: ax1 = fig.add_subplot(211)
In [158]: ax2 = fig.add_axes([0.1, 0.1, 0.7, 0.3])
In [159]: ax1
Out[159]: <Axes:>
In [160]: print(fig.axes)
[<Axes:>, <matplotlib.axes._axes.Axes object at 0x7f0768702be0>]
因为 figure 维护“当前 Axes”的概念(请参阅 Figure.gca
和 Figure.sca
)以支持 pylab/pyplot 状态机,所以您不应直接从 Axes 列表中插入或删除 Axes,而应使用 add_subplot()
和 add_axes()
方法插入,并使用 Axes.remove
方法删除。但是,您可以自由地迭代 Axes 列表或索引到列表中以访问要自定义的 Axes
实例。这是一个将所有 Axes 网格打开的示例
figure 还具有自己的 images
、lines
、patches
和 text
属性,您可以使用它们直接添加基本对象。这样做时,Figure
的默认坐标系将仅使用像素(通常不是您想要的)。如果改为使用 Figure 级别的方法来添加 Artists(例如,使用 Figure.text
添加文本),则默认坐标系将为“figure 坐标”,其中 (0, 0) 是图形的左下角,(1, 1) 是图形的右上角。
与所有 Artist
一样,您可以通过设置 transform 属性来控制此坐标系。您可以通过将 Artist
转换设置为 fig.transFigure
来显式使用“figure 坐标”
import matplotlib.lines as lines
fig = plt.figure()
l1 = lines.Line2D([0, 1], [0, 1], transform=fig.transFigure, figure=fig)
l2 = lines.Line2D([0, 1], [1, 0], transform=fig.transFigure, figure=fig)
fig.lines.extend([l1, l2])
plt.show()
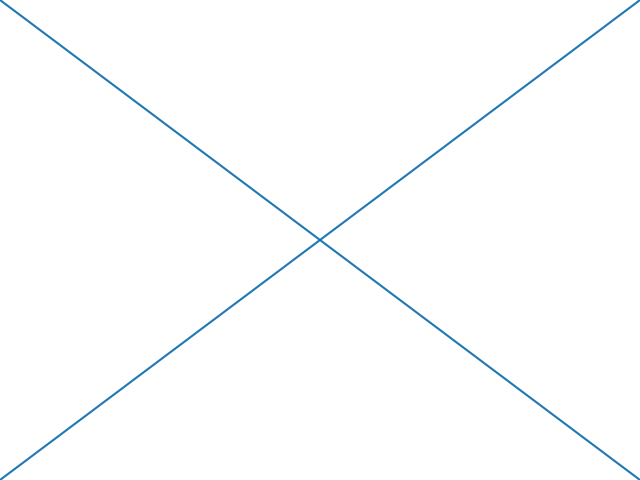
以下是 Figure 包含的 Artists 的摘要
Axes 容器#
matplotlib.axes.Axes
是 Matplotlib 世界的核心 —— 它包含了图中绝大多数使用的 Artist
,并提供了许多辅助方法来创建这些 Artist
并添加到自身,以及访问和自定义其包含的 Artist
的辅助方法。与 Figure
类似,它包含一个 Patch
matplotlib.axes.Axes.patch
,对于笛卡尔坐标系,它是一个 Rectangle
,对于极坐标系,它是一个 Circle
;这个 patch 决定了绘图区域的形状、背景和边框。
ax = fig.add_subplot()
rect = ax.patch # a Rectangle instance
rect.set_facecolor('green')
当你调用绘图方法时,例如典型的 plot
并传入数组或值列表时,该方法将创建一个 matplotlib.lines.Line2D
实例,使用作为关键字参数传递的所有 Line2D
属性更新该线条,将该线条添加到 Axes
,然后将其返回给你。
plot
返回一个线条列表,因为你可以传入多个 x、y 对进行绘制,我们将长度为 1 的列表的第一个元素解包到 line 变量中。该线条已被添加到 Axes.lines
列表中。
In [229]: print(ax.lines)
[<matplotlib.lines.Line2D at 0xd378b0c>]
类似地,创建 patch 的方法,例如 bar()
创建一个矩形列表,会将这些 patch 添加到 Axes.patches
列表中。
你不应该直接将对象添加到 Axes.lines
或 Axes.patches
列表中,因为 Axes
在创建和添加对象时需要执行一些操作。
它设置
Artist
的figure
和axes
属性;它设置默认的
Axes
变换(除非已设置);它检查
Artist
中包含的数据,以更新控制自动缩放的数据结构,以便可以调整视图限制以包含绘制的数据。
但是,你可以自己创建对象,并使用诸如 add_line
和 add_patch
之类的辅助方法直接将它们添加到 Axes
中。以下是一个带注释的交互式会话,说明正在发生的事情。
In [262]: fig, ax = plt.subplots()
# create a rectangle instance
In [263]: rect = matplotlib.patches.Rectangle((1, 1), width=5, height=12)
# by default the Axes instance is None
In [264]: print(rect.axes)
None
# and the transformation instance is set to the "identity transform"
In [265]: print(rect.get_data_transform())
IdentityTransform()
# now we add the Rectangle to the Axes
In [266]: ax.add_patch(rect)
# and notice that the ax.add_patch method has set the Axes
# instance
In [267]: print(rect.axes)
Axes(0.125,0.1;0.775x0.8)
# and the transformation has been set too
In [268]: print(rect.get_data_transform())
CompositeGenericTransform(
TransformWrapper(
BlendedAffine2D(
IdentityTransform(),
IdentityTransform())),
CompositeGenericTransform(
BboxTransformFrom(
TransformedBbox(
Bbox(x0=0.0, y0=0.0, x1=1.0, y1=1.0),
TransformWrapper(
BlendedAffine2D(
IdentityTransform(),
IdentityTransform())))),
BboxTransformTo(
TransformedBbox(
Bbox(x0=0.125, y0=0.10999999999999999, x1=0.9, y1=0.88),
BboxTransformTo(
TransformedBbox(
Bbox(x0=0.0, y0=0.0, x1=6.4, y1=4.8),
Affine2D(
[[100. 0. 0.]
[ 0. 100. 0.]
[ 0. 0. 1.]])))))))
# the default Axes transformation is ax.transData
In [269]: print(ax.transData)
CompositeGenericTransform(
TransformWrapper(
BlendedAffine2D(
IdentityTransform(),
IdentityTransform())),
CompositeGenericTransform(
BboxTransformFrom(
TransformedBbox(
Bbox(x0=0.0, y0=0.0, x1=1.0, y1=1.0),
TransformWrapper(
BlendedAffine2D(
IdentityTransform(),
IdentityTransform())))),
BboxTransformTo(
TransformedBbox(
Bbox(x0=0.125, y0=0.10999999999999999, x1=0.9, y1=0.88),
BboxTransformTo(
TransformedBbox(
Bbox(x0=0.0, y0=0.0, x1=6.4, y1=4.8),
Affine2D(
[[100. 0. 0.]
[ 0. 100. 0.]
[ 0. 0. 1.]])))))))
# notice that the xlimits of the Axes have not been changed
In [270]: print(ax.get_xlim())
(0.0, 1.0)
# but the data limits have been updated to encompass the rectangle
In [271]: print(ax.dataLim.bounds)
(1.0, 1.0, 5.0, 12.0)
# we can manually invoke the auto-scaling machinery
In [272]: ax.autoscale_view()
# and now the xlim are updated to encompass the rectangle, plus margins
In [273]: print(ax.get_xlim())
(0.75, 6.25)
# we have to manually force a figure draw
In [274]: fig.canvas.draw()
有许多 Axes
辅助方法用于创建原始的 Artist
并将它们添加到各自的容器中。下表总结了其中的一小部分,它们创建的 Artist
类型以及它们存储的位置。
Axes 辅助方法 |
Artist |
容器 |
---|---|---|
|
ax.texts |
|
|
ax.patches |
|
|
ax.lines 和 ax.patches |
|
|
ax.patches |
|
|
ax.patches |
|
|
ax.images |
|
|
ax.get_legend() |
|
|
ax.lines |
|
|
ax.collections |
|
|
ax.texts |
除了所有这些 Artist
之外,Axes
还包含两个重要的 Artist
容器:XAxis
和 YAxis
,它们负责绘制刻度和标签。它们存储为实例变量 matplotlib.axes.Axes.xaxis
和 matplotlib.axes.Axes.yaxis
。XAxis
和 YAxis
容器将在下面详细介绍,但请注意,Axes
包含许多辅助方法,这些方法将调用转发到 Axis
实例,因此除非你想直接使用它们,否则通常不需要直接使用它们。例如,你可以使用 Axes
辅助方法设置 XAxis
刻度标签的字体颜色。
下面总结了 Axes
包含的 Artists。
Axes 属性 |
描述 |
---|---|
artists |
|
patch |
|
collections |
|
images |
|
lines |
|
patches |
|
texts |
|
xaxis |
|
yaxis |
可以通过 get_legend
访问图例。
轴容器#
matplotlib.axis.Axis
实例负责处理刻度线、网格线、刻度标签和轴标签的绘制。您可以分别配置 y 轴的左侧和右侧刻度,以及 x 轴的上方和下方刻度。Axis
还存储自动缩放、平移和缩放中使用的数据和视图间隔,以及控制刻度位置和如何表示为字符串的 Locator
和 Formatter
实例。
每个 Axis
对象都包含一个 label
属性(这是 pyplot
在调用 xlabel
和 ylabel
时修改的内容),以及一个主刻度和次刻度列表。刻度是 axis.XTick
和 axis.YTick
实例,其中包含呈现刻度和刻度标签的实际线和文本基元。由于刻度是根据需要动态创建的(例如,在平移和缩放时),因此您应该通过它们的访问器方法 axis.Axis.get_major_ticks
和 axis.Axis.get_minor_ticks
访问主刻度和次刻度列表。尽管刻度包含所有基元,并且将在下面介绍,但 Axis
实例具有返回刻度线、刻度标签、刻度位置等的访问器方法。
fig, ax = plt.subplots()
axis = ax.xaxis
axis.get_ticklocs()
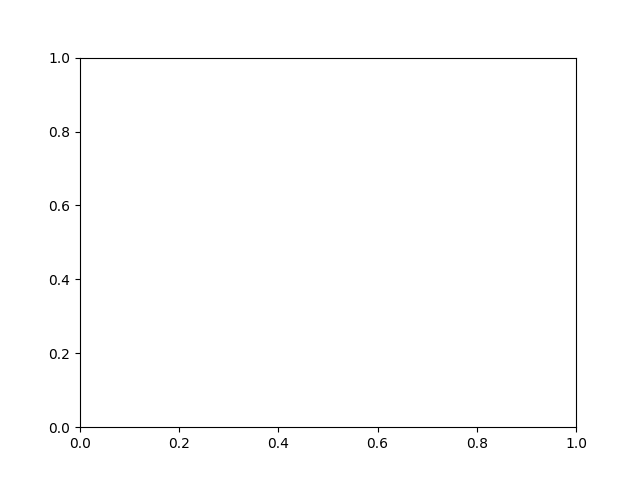
array([0. , 0.2, 0.4, 0.6, 0.8, 1. ])
[Text(0.0, 0, '0.0'), Text(0.2, 0, '0.2'), Text(0.4, 0, '0.4'), Text(0.6000000000000001, 0, '0.6'), Text(0.8, 0, '0.8'), Text(1.0, 0, '1.0')]
注意,刻度线的数量是标签的两倍,因为默认情况下,顶部和底部都有刻度线,但只有 x 轴下方有刻度标签;但是,这是可以自定义的。
<a list of 12 Line2D ticklines objects>
通过上述方法,默认情况下您只会得到主刻度列表,但您也可以请求次刻度。
axis.get_ticklabels(minor=True)
axis.get_ticklines(minor=True)
<a list of 0 Line2D ticklines objects>
以下是 Axis
的一些有用的访问器方法的摘要(这些方法在有用的情况下具有相应的设置器,例如 set_major_formatter()
。)
轴访问器方法 |
描述 |
---|---|
轴的刻度,例如“log”或“linear” |
|
轴视图限制的间隔实例 |
|
轴数据限制的间隔实例 |
|
轴的网格线列表 |
|
轴标签 - 一个 |
|
轴偏移文本 - 一个 |
|
一个 |
|
一个 |
|
刻度位置列表 - 关键字 minor=True|False |
|
主刻度的 |
|
主刻度的 |
|
次刻度的 |
|
次刻度的 |
|
主刻度的 |
|
次刻度的 |
|
打开或关闭主刻度或次刻度的网格 |
这是一个示例,不推荐其美观性,它自定义了轴和刻度属性。
# plt.figure creates a matplotlib.figure.Figure instance
fig = plt.figure()
rect = fig.patch # a rectangle instance
rect.set_facecolor('lightgoldenrodyellow')
ax1 = fig.add_axes([0.1, 0.3, 0.4, 0.4])
rect = ax1.patch
rect.set_facecolor('lightslategray')
for label in ax1.xaxis.get_ticklabels():
# label is a Text instance
label.set_color('red')
label.set_rotation(45)
label.set_fontsize(16)
for line in ax1.yaxis.get_ticklines():
# line is a Line2D instance
line.set_color('green')
line.set_markersize(25)
line.set_markeredgewidth(3)
plt.show()
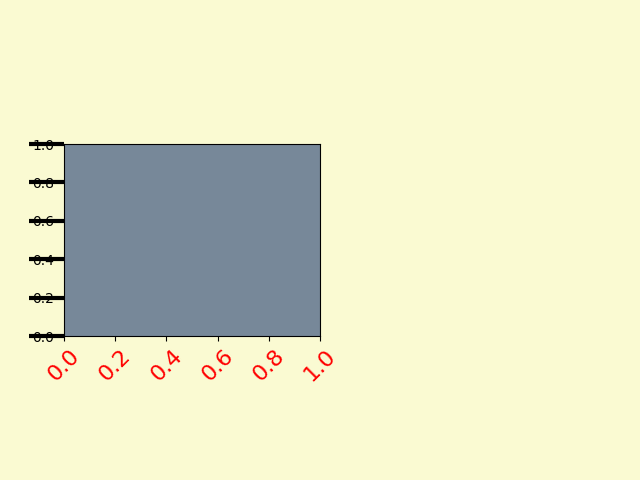
刻度容器#
matplotlib.axis.Tick
是我们从 Figure
到 Axes
到 Axis
到 Tick
的最终容器对象。Tick
包含刻度线和网格线实例,以及上下刻度的标签实例。这些都可以直接作为 Tick
的属性进行访问。
刻度属性 |
描述 |
---|---|
tick1line |
一个 |
tick2line |
一个 |
gridline |
一个 |
label1 |
一个 |
label2 |
一个 |
这是一个示例,它使用美元符号设置右侧刻度的格式化程序,并在 y 轴右侧将其颜色设置为绿色。
import matplotlib.pyplot as plt
import numpy as np
# Fixing random state for reproducibility
np.random.seed(19680801)
fig, ax = plt.subplots()
ax.plot(100*np.random.rand(20))
# Use automatic StrMethodFormatter
ax.yaxis.set_major_formatter('${x:1.2f}')
ax.yaxis.set_tick_params(which='major', labelcolor='green',
labelleft=False, labelright=True)
plt.show()
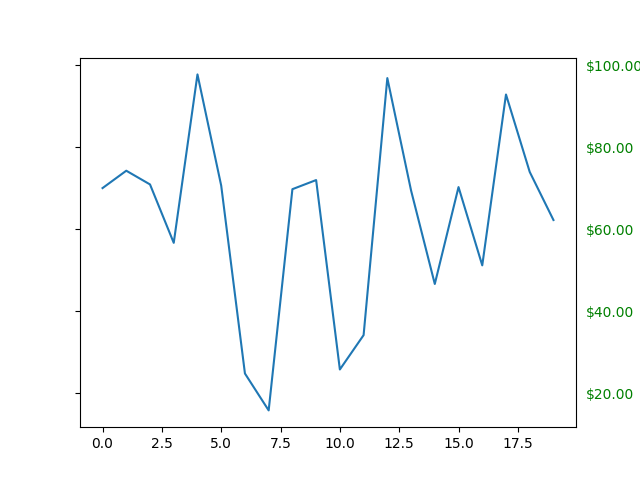
脚本的总运行时间:(0 分钟 1.178 秒)